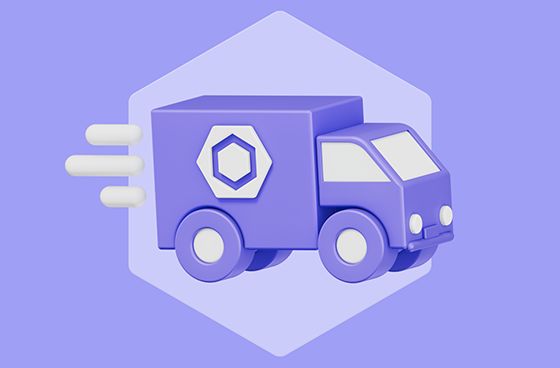
Highlights
This is a summary of the significant changes, both breaking and non-breaking, you need to know about when upgrading from ESLint v8.x to ESLint v9.0.0.
Installing
Because this is a major release, you may not automatically be upgraded by npm. To ensure you are using this version, run:
npm i eslint@9.0.0 --save-dev
Migration Guide
As there are a lot of changes, we’ve created a migration guide describing the breaking changes in great detail along with the steps you should take to address them. We expect that most users should be able to upgrade without any build changes, but the migration guide should be a useful resource if you encounter problems.
Node.js < v18.18.0, v19 no longer supported
As of this post, Node.js v20.x is the LTS release, and as such we are dropping support for all versions of Node.js prior to v18.18.0 as well as v19.x.
Flat config is now the default and has some changes
Flat config is now the default configuration format for ESLint and eslintrc is officially deprecated. To continue using a eslintrc configuration file, you’ll need to set the ESLINT_USE_FLAT_CONFIG
environment variable to false
.
This change affects users, plugin developers, and integrators as many aspects of ESLint had to change to make this happen. Please see our previous blog post for more details.
This release also introduces the config inspector, which can be launched on the command line using --inspect-config
.
Removed all formatters except stylish
, html
, json
, and json-with-meta
The following formatters have been removed:
checkstyle
compact
jslint-xml
junit
tap
unix
visualstudio
If you are using these formatters currently, you’ll need to install the standalone packages for use with ESLint v9.0.0.
Removed valid-jsdoc
and require-jsdoc
rules
We have removed valid-jsdoc
and require-jsdoc
. We recommend using the eslint-plugin-jsdoc
plugin instead.
Removed deprecated methods on context
and SourceCode
As we announced in September, we have removed a lot of deprecated methods from context
and replaced them with methods on SourceCode
.
Updated eslint:recommended
The eslint:recommended
configuration is updated to include new rules that we feel are important, and to remove deprecated and less important rules.
New rule: no-useless-assignment
ESLint v9.0.0 introduces a new rule, no-useless-assignment
, that is designed to catch situations where you’ve assigned a value to a variable and that value is never used. For example:
let id = 1234; // 1234 is never used
id = calculateId();
Updates to existing rules
- The
complexity
rule now also takes into account optional chaining and default values in destructuring patterns and parameters. - The
no-fallthrough
rule has a new optionreportUnusedFallthroughComment
. - The
no-inner-declarations
rule has a new default behavior. In v8.x, the rule would flag any functions defined inside of blocks as errors because this behavior was undefined in early versions of JavaScript. As of ES 2015, block-scoped function declarations are well-defined and so we changed the default behavior to not warn on block-scoped functions. - The
no-misleading-character-class
rule now highlights the offending characters in a regular expression rather than the entire regular expression. - The
no-restricted-imports
rule changed the behavior ofpaths
. In v8.x, if multiple entries in thepaths
array of your configuration for Theno-restricted-imports
rule had the samename
property, only the last one would apply. In v9.0.0, all entries apply, allowing for specifying different error messages for different imported names. - The
no-restricted-imports
rule has new optionsallowImportNames
andallowImportNamePattern
. - The
no-unused-vars
rulevarsIgnorePattern
option no longer applies to caught error variables. - The
no-unused-vars
rule has a new default value for thecaughtErrors
option (changed from"none"
to"all"
). - The
no-unused-vars
rule has a new optionignoreClassWithStaticInitBlock
. - The
no-unused-vars
rule has a new optionreportUsedIgnorePattern
. - The
no-useless-computed-key
rule has a new default value for theenforceForClassMembers
option (changed fromfalse
totrue
). This is intended to help avoiding misleading comments that can occur as a result of refactoring. When this option is set totrue
, the rule will prohibit a fallthrough comment if a case can never fallthrough.
New API loadESLint()
ESLint now exports a new function loadESLint()
from its main entry point. Integrations can use this function to get either the ESLint
class (former FlatESLint
class) or the LegacyESLint
class (former ESLint
class) and thus easily swap between flat config and eslintrc APIs.
Changes to how you write rules
We’ve made multiple changes to help prevent errors in rules:
- Function-style rules will stop working in v9.0.0. Function-style rules are rules created by exporting a function from a file rather than exporting an object with a
create()
method. - When a rule doesn’t have
meta.schema
specified, a default schema of[]
will be applied. This means that rules without a schema will be assumed to have no options, which in turn means that validation will fail if options are provided.
Stricter RuleTester
validations
This release adds more checks in RuleTester
:
- Messages cannot have unsubstituted placeholders.
- Suggestions must change the code.
- Suggestion messages must be unique for the same lint problem.
- Suggestions must generate valid syntax.
- Test case
output
must be different fromcode
. - Test error objects must specify
message
ormessageId
. - Test error object must specify
suggestions
if the actual error provides suggestions. - Test suggestion objects must specify
desc
ormessageId
. - Test suggestion objects must specify
output
. filename
andonly
properties of test objects must be of the expected type (string
andboolean
, respectively).- Duplicate tests cause an error.
The --output-file
flag now guarantees a file is output
The --output-file
CLI flag is designed to output the results of the ESLint run to specified file. Prior to this release, no file would be output if linting passed with no errors or warnings. In v9.0.0, an empty file will be output when linting passes without any errors or warnings.
Better scope analysis
In v9.0.0, we updated the behavior of eslint-scope
to fix a couple of longstanding bugs:
- Previously, ESLint would treat
("use strict")
as a strict mode directive even though it is not. We fixed the behavior so only valid strict mode directives are honored. - The containing scope of a class
extends
clause was incorrectly set to be the scope containing the class when it should have been the class scope itself. This has been fixed.
CodePath#currentSegments
removed
As announced in our previous post, CodePath#currentSegments
has been removed from the rules API. Please refer to the post for more details.
Precalculated Code Paths
ESLint v9.0.0 now precalculates code path information before the traversal used by rules. As a result, the code path information is now complete regardless of where it is accessed inside of a rule.
Multiple /* eslint */
comments for the same rule are now disallowed
In ESLint v8.x, if the file being linted contained multiple /* eslint */
configuration comments for the same rule, the last one would be applied, while the others would be silently ignored.
In ESLint v9.0.0, the first one is applied, while the others are reported as lint errors.
--quiet
option is more performant
The --quiet
option hides all warnings in the ESLint console. In v9.0.0, we are making a performance improvement by also not executing any rules set to "warn"
.
Running eslint
with no file arguments
If you are using flat config and you don’t pass any file arguments to the CLI, the CLI will default to linting the current directory, which means you can type npx eslint
and it will just work. (Doing the same with an eslintrc config file will result in an error.)
Unused disable directives cause warnings by default
ESLint has long been able to flag unused disable directives. In this release, we’ve enabled warnings for unused disable directives by default. You can modify this value in your config file with linterOptions.reportUnusedDisableDirectives
or on the command line using --report-unused-disable-directives-severity
.
Performance statistics available in formatters via --stats
The information from the rule profiler is now available inside of formatters when the --stats
flag is used in the CLI. This allows anyone to create custom visualizations of the performance information that ESLint tracks.
Breaking Changes
b7cf3bd
fix!: correctcamelcase
rule schema forallow
option (#18232) (eMerzh)09bd7fe
feat!: move AST traversal into SourceCode (#18167) (Nicholas C. Zakas)79a95eb
feat!: disallow multiple configuration comments for same rule (#18157) (Milos Djermanovic)9163646
feat!: Rule Tester checks for missing placeholder data in the message (#18073) (fnx)3c4d51d
feat!: default forenforceForClassMembers
inno-useless-computed-key
(#18054) (Francesco Trotta)47e60f8
feat!: Stricter rule test validations (#17654) (fnx)1a94589
feat!:no-unused-vars
default caughtErrors to ‘all’ (#18043) (Josh Goldberg ✨)57089cb
feat!: no-restricted-imports allow multiple config entries for same path (#18021) (Milos Djermanovic)2e1d549
feat!: detect duplicate test cases (#17955) (Bryan Mishkin)701f1af
feat!: no-inner-declaration new default behaviour and option (#17885) (Tanuj Kanti)bde5105
fix!: handle--output-file
for empty output when saving to disk (#17957) (Nitin Kumar)07107a5
fix!: upgrade eslint-scope@8.0.0 (#17942) (Milos Djermanovic)3ee0f6c
fix!: no-unused-varsvarsIgnorePattern
behavior with catch arguments (#17932) (Tanuj Kanti)51f8bc8
fix!: configuration comments with just severity should retain options (#17945) (Milos Djermanovic)d191bdd
feat!: Remove CodePath#currentSegments (#17936) (Milos Djermanovic)946ae00
feat!: FlatRuleTester -> RuleTester (#17922) (Nicholas C. Zakas)baff28c
feat!: removeno-inner-declarations
fromeslint:recommended
(#17920) (Milos Djermanovic)cadfbcd
feat!: Rename FlatESLint to ESLint (#17914) (Nicholas C. Zakas)d1018fc
feat!: skip running warnings in --quiet mode (#17274) (Maddy Miller)fb81b1c
feat!: Set defaultschema: []
, drop support for function-style rules (#17792) (Milos Djermanovic)0b21e1f
feat!: add two more cases tono-implicit-coercion
(#17832) (Gürgün Dayıoğlu)2916c63
feat!: Switch Linter to flat config by default (#17851) (Nicholas C. Zakas)200518e
fix!: Parsing ‘exported’ comment using parseListConfig (#17675) (amondev)bdd6ba1
feat!: Remove valid-jsdoc and require-jsdoc (#17694) (Nicholas C. Zakas)12be307
fix!: Behavior of CLI when no arguments are passed (#17644) (Nicholas C. Zakas)8fe8c56
feat!: Update shouldUseFlatConfig and CLI so flat config is default (#17748) (Nicholas C. Zakas)60dea3e
feat!: deprecate no-new-symbol, recommend no-new-native-nonconstructor (#17710) (Francesco Trotta)5aa9c49
feat!: check for parsing errors in suggestion fixes (#16639) (Bryan Mishkin)b3e0bb0
feat!: assert suggestion messages are unique in rule testers (#17532) (Josh Goldberg ✨)e563c52
feat!:no-invalid-regexp
make allowConstructorFlags case-sensitive (#17533) (Josh Goldberg ✨)e5f02c7
fix!: no-sequences rule schema correction (#17878) (MHO)6ee3e9e
feat!: Updateeslint:recommended
configuration (#17716) (Milos Djermanovic)c2cf85a
feat!: drop support for string configurations in flat config array (#17717) (Milos Djermanovic)c314fd6
feat!: RemoveSourceCode#getComments()
(#17715) (Milos Djermanovic)ae78ff1
feat!: Remove deprecated context methods (#17698) (Nicholas C. Zakas)f71c328
feat!: Swap FlatESLint-ESLint, FlatRuleTester-RuleTester in API (#17823) (Nicholas C. Zakas)5304da0
feat!: remove formatters except html, json(-with-metadata), and stylish (#17531) (Josh Goldberg ✨)e1e827f
feat!: Require Node.js^18.18.0 || ^20.9.0 || >=21.1.0
(#17725) (Milos Djermanovic)
Features
d54a412
feat: Add --inspect-config CLI flag (#18270) (Nicholas C. Zakas)97ce45b
feat: AddreportUsedIgnorePattern
option tono-unused-vars
rule (#17662) (Pearce Ropion)3e9fcea
feat: Show config names in error messages (#18256) (Nicholas C. Zakas)de40874
feat: Rule Performance Statistics for flat ESLint (#17850) (Mara Kiefer)d85c436
feat: use-isnan report NaN inindexOf
andlastIndexOf
with fromIndex (#18225) (Tanuj Kanti)b8fb572
feat: addreportUnusedFallthroughComment
option to no-fallthrough rule (#18188) (Kirk Waiblinger)1c173dc
feat: addignoreClassWithStaticInitBlock
option tono-unused-vars
(#18170) (Tanuj Kanti)a451b32
feat: makeno-misleading-character-class
report more granular errors (#18082) (Francesco Trotta)c49ed63
feat: update complexity rule for optional chaining & default values (#18152) (Mathias Schreck)11144a2
feat:no-restricted-imports
option addedallowImportNames
(#16196) (M Pater)74124c2
feat: add suggestions touse-isnan
inindexOf
&lastIndexOf
calls (#18063) (StyleShit)53f0f47
feat: Add loadESLint() API method for v9 (#18097) (Nicholas C. Zakas)2d11d46
feat: add suggestions touse-isnan
in binary expressions (#17996) (StyleShit)26093c7
feat: fix false negatives inno-this-before-super
(#17762) (Yosuke Ota)5471e43
feat: convert unsafe autofixes to suggestions inno-implicit-coercion
(#17985) (Gürgün Dayıoğlu)e3051be
feat: emit warning when.eslintignore
file is detected (#17952) (Nitin Kumar)a630edd
feat: maintain latest ecma version in ESLint (#17958) (Milos Djermanovic)b4e0503
feat: addno-useless-assignment
rule (#17625) (Yosuke Ota)287c4b7
feat:no-misleading-character-class
granular errors (#17515) (Josh Goldberg ✨)8792464
feat: Enable eslint.config.mjs and eslint.config.cjs (#17909) (Nicholas C. Zakas)24ce927
feat: warn by default for unused disable directives (#17879) (Bryan Mishkin)
Bug Fixes
610c148
fix: Supportusing
declarations in no-lone-blocks (#18269) (Kirk Waiblinger)e508800
fix: rule tester ignore irrelevant test case properties (#18235) (fnx)a129acb
fix: flat config name on ignores object (#18258) (Nicholas C. Zakas)dadc5bf
fix:constructor-super
false positives with loops (#18226) (Milos Djermanovic)ae8103d
fix: load plugins in the CLI in flat config mode (#18185) (Francesco Trotta)e37153f
fix: improve error message for invalid rule config (#18147) (Nitin Kumar)af6e170
fix: stop linting files after an error (#18155) (Francesco Trotta)0cb4914
fix: validate options when comment with just severity enables rule (#18133) (Milos Djermanovic)c4d26fd
fix:use-isnan
doesn’t report onSequenceExpression
s (#18059) (StyleShit)39076fb
fix: handle absolute file paths inRuleTester
(#17989) (Nitin Kumar)6d11f3d
fix: Ensure config keys are printed for config errors (#17980) (Nicholas C. Zakas)806f708
fix:no-misleading-character-class
edge cases with granular errors (#17970) (Milos Djermanovic)f182114
fix: deep merge behavior in flat config (#17906) (Francesco Trotta)b577e8a
fix: allow circular references in config (#17752) (Francesco Trotta)
Documentation
e151050
docs: update get-started to the new@eslint/create-config
(#18217) (唯然)94178ad
docs: mention aboutname
field in flat config (#18252) (Anthony Fu)1765c24
docs: add Troubleshooting page (#18181) (Josh Goldberg ✨)96607d0
docs: version selectors synchronization (#18260) (Milos Djermanovic)651ec91
docs: remove/* eslint-env */
comments from rule examples (#18249) (Milos Djermanovic)950c4f1
docs: Update README (GitHub Actions Bot)12f5746
docs: add info about dot files and dir in flat config (#18239) (Tanuj Kanti)b93f408
docs: update shared settings example (#18251) (Tanuj Kanti)26384d3
docs: fixecmaVersion
in one example, add checks (#18241) (Milos Djermanovic)7747097
docs: Update PR review process (#18233) (Nicholas C. Zakas)b07d427
docs: fix typo (#18246) (Kirill Gavrilov)778082d
docs: add Glossary page (#18187) (Josh Goldberg ✨)239a7e2
docs: Clarify the description ofsort-imports
options (#18198) (gyeongwoo park)4769c86
docs: fix incorrect example inno-lone-blocks
(#18215) (Tanuj Kanti)5251327
docs: Update README (GitHub Actions Bot)1dc8618
docs: Update README (GitHub Actions Bot)ba1c1bb
docs: Update README (GitHub Actions Bot)337cdf9
docs: Explain limitations of RuleTester fix testing (#18175) (Nicholas C. Zakas)c7abd89
docs: Explain Node.js version support (#18176) (Nicholas C. Zakas)d961eeb
docs: show red underlines in examples in rules docs (#18041) (Yosuke Ota)558274a
docs: Update README (GitHub Actions Bot)2908b9b
docs: Update release documentation (#18174) (Nicholas C. Zakas)1f1260e
docs: replace HackerOne link with GitHub advisory (#18165) (Francesco Trotta)e5ef3cd
docs: add inline cases condition inno-fallthrough
(#18158) (Tanuj Kanti)450d0f0
docs: fixignore
option docs (#18154) (Francesco Trotta)5fe095c
docs: show v8.57.0 as latest version in dropdown (#18142) (Milos Djermanovic)7db5bb2
docs: Show prerelease version in dropdown (#18135) (Nicholas C. Zakas)73a5f06
docs: Update README (GitHub Actions Bot)f95cd27
docs: Disallow multiple rule configuration comments in the same example (#18116) (Milos Djermanovic)d8068ec
docs: Update link for schema examples (#18112) (Svetlana)f1c7e6f
docs: Switch to Ethical Ads (#18090) (Strek)15c143f
docs: JS Foundation -> OpenJS Foundation in PR template (#18092) (Nicholas C. Zakas)6ea339e
docs: add stricter rule test validations to v9 migration guide (#18085) (Milos Djermanovic)3c816f1
docs: use relative link from CLI to core concepts (#18083) (Milos Djermanovic)9458735
docs: fix malformedeslint
config comments in rule examples (#18078) (Francesco Trotta)07a1ada
docs: link from--fix
CLI doc to the relevant core concept (#18080) (Bryan Mishkin)b844324
docs: Update team responsibilities (#18048) (Nicholas C. Zakas)aadfb60
docs: document languageOptions and other v9 changes for context (#18074) (fnx)857e242
docs: tweak explanation for meta.docs rule properties (#18057) (Bryan Mishkin)10485e8
docs: recommend messageId over message for reporting rule violations (#18050) (Bryan Mishkin)98b5ab4
docs: Update README (GitHub Actions Bot)505fbf4
docs: updateno-restricted-imports
rule (#18015) (Tanuj Kanti)c25b4af
docs: Update README (GitHub Actions Bot)33d1ab0
docs: add more examples to flat config ignores docs (#18020) (Milos Djermanovic)e6eebca
docs: Update sort-keys options properties count (#18025) (LB (Ben Johnston))1fedfd2
docs: Improve flat config ignores docs (#17997) (Nicholas C. Zakas)38b9b06
docs: update valid-typeof rule (#18001) (Tanuj Kanti)b4abfea
docs: Update note about ECMAScript support (#17991) (Francesco Trotta)6788873
docs: Update release blog post template (#17994) (Nicholas C. Zakas)1f37442
docs: Add sections on non-npm plugin configuration (#17984) (Nicholas C. Zakas)96307da
docs: migration guide entry forno-inner-declarations
(#17977) (Tanuj Kanti)40be60e
docs: Update README (GitHub Actions Bot)d31c180
docs: fix number of code-path events on custom rules page (#17969) (Richard Hunter)1529ab2
docs: reorder entries in v9 migration guide (#17967) (Milos Djermanovic)9507525
docs: Explain how to combine configs (#17947) (Nicholas C. Zakas)7c78576
docs: Add more removedcontext
methods to migrate to v9 guide (#17951) (Milos Djermanovic)3a877d6
docs: Update removed CLI flags migration (#17939) (Nicholas C. Zakas)4a9cd1e
docs: Update Linter API for v9 (#17937) (Milos Djermanovic)2a8eea8
docs: update docs for v9.0.0-alpha.0 (#17929) (Milos Djermanovic)7f0ba51
docs: showNEXT
in version selectors (#17911) (Milos Djermanovic)0a7911e
docs: add flat config default to v9 migration guide (#17927) (Milos Djermanovic)94f8065
docs: Add CLI updates to migrate to v9 guide (#17924) (Nicholas C. Zakas)16187f2
docs: Add exported and string config notes to migrate to v9 guide (#17926) (Nicholas C. Zakas)3ae50cc
docs: Add RuleTester changes to migrate to v9 guide (#17923) (Nicholas C. Zakas)0831b58
docs: add rule changes to v9 migration guide (#17925) (Milos Djermanovic)037abfc
docs: update API docs (#17919) (Milos Djermanovic)afc3c03
docs: add function-style andmeta.schema
changes to v9 migration guide (#17912) (Milos Djermanovic)1da0723
docs: updateeslint:recommended
section in Migrate to v9.x (#17908) (Milos Djermanovic)f55881f
docs: remove configuration-files-new.md (#17907) (Milos Djermanovic)63ae191
docs: Migrate to v9.0.0 (#17905) (Nicholas C. Zakas)e708496
docs: Switch to flat config by default (#17840) (Nicholas C. Zakas)fdf0424
docs: Update Create a Plugin for flat config (#17826) (Nicholas C. Zakas)e6a91bd
docs: Switch shareable config docs to use flat config (#17827) (Nicholas C. Zakas)3831fb7
docs: updated examples ofmax-lines
rule (#17898) (Tanuj Kanti)cd1ac20
docs: Update README (GitHub Actions Bot)
Build Related
26010c2
Build: changelog update for 9.0.0-rc.0 (Jenkins)b91f9dc
build: fix TypeError in prism-eslint-hooks.js (#18209) (Francesco Trotta)d7ec0d1
Build: changelog update for 9.0.0-beta.2 (Jenkins)fd9c0a9
Build: changelog update for 9.0.0-beta.1 (Jenkins)c9f2f33
build: changelog update for 8.57.0 (#18144) (Milos Djermanovic)1bbc495
Build: changelog update for 9.0.0-beta.0 (Jenkins)96f8877
Build: changelog update for 9.0.0-alpha.2 (Jenkins)52d5e7a
Build: changelog update for 9.0.0-alpha.1 (Jenkins)c2bf27d
build: update docs files when publishing prereleases (#17940) (Milos Djermanovic)e91d85d
Build: changelog update for 9.0.0-alpha.0 (Jenkins)
Chores
19f9a89
chore: Update dependencies for v9.0.0 (#18275) (Nicholas C. Zakas)7c957f2
chore: package.json update for @eslint/js release (Jenkins)d73a33c
chore: ignore/docs/v8.x
in link checker (#18274) (Milos Djermanovic)44a81c6
chore: upgrade knip (#18272) (Lars Kappert)e80b60c
chore: remove code for testing version selectors (#18266) (Milos Djermanovic)a98babc
chore: add npm script to run WebdriverIO test (#18238) (Francesco Trotta)9b7bd3b
chore: update dependency markdownlint to ^0.34.0 (#18237) (renovate[bot])297416d
chore: package.json update for eslint-9.0.0-rc.0 (#18223) (Francesco Trotta)d363c51
chore: package.json update for @eslint/js release (Jenkins)1b841bb
chore: fix some comments (#18213) (avoidaway)29c3595
chore: remove repetitive words (#18193) (cuithon)acc2e06
chore: Introduce Knip (#18005) (Lars Kappert)7509276
chore: upgrade @eslint/js@9.0.0-beta.2 (#18180) (Milos Djermanovic)96087b3
chore: package.json update for @eslint/js release (Jenkins)925afa2
chore: Remove some uses oflodash.merge
(#18179) (Milos Djermanovic)972ef15
chore: remove invalid type in @eslint/js (#18164) (Nitin Kumar)32ffdd1
chore: upgrade @eslint/js@9.0.0-beta.1 (#18146) (Milos Djermanovic)e41425b
chore: package.json update for @eslint/js release (Jenkins)bb3b9c6
chore: upgrade @eslint/eslintrc@3.0.2 (#18145) (Milos Djermanovic)e462524
chore: upgrade eslint-release@3.2.2 (#18138) (Milos Djermanovic)8e13a6b
chore: fix spelling mistake in README.md (#18128) (Will Eastcott)66f52e2
chore: remove unused tools rule-types.json, update-rule-types.js (#18125) (Josh Goldberg ✨)bf0c7ef
ci: fix sync-labels value of pr-labeler (#18124) (Tanuj Kanti)cace6d0
ci: add PR labeler action (#18109) (Nitin Kumar)1a65d3e
chore: exportbase
config fromeslint-config-eslint
(#18119) (Milos Djermanovic)9aa4df3
refactor: removeglobals
dependency (#18115) (Milos Djermanovic)e40d1d7
chore: upgrade @eslint/js@9.0.0-beta.0 (#18108) (Milos Djermanovic)9870f93
chore: package.json update for @eslint/js release (Jenkins)2c62e79
chore: upgrade @eslint/eslintrc@3.0.1 (#18107) (Milos Djermanovic)81f0294
chore: upgrade espree@10.0.1 (#18106) (Milos Djermanovic)5e2b292
chore: upgrade eslint-visitor-keys@4.0.0 (#18105) (Milos Djermanovic)ce838ad
chore: replace dependency npm-run-all with npm-run-all2 ^5.0.0 (#18045) (renovate[bot])54df731
chore: update dependency markdownlint-cli to ^0.39.0 (#18084) (renovate[bot])8f06a60
chore: update dependency shelljs to ^0.8.5 (#18079) (Francesco Trotta)93ffe30
chore: update dependency file-entry-cache to v8 (#17903) (renovate[bot])6ffdcbb
chore: upgrade @eslint/js@9.0.0-alpha.2 (#18038) (Milos Djermanovic)2c12715
chore: package.json update for @eslint/js release (Jenkins)cc74c4d
chore: upgrade espree@10.0.0 (#18037) (Milos Djermanovic)dfb68b6
chore: use Node.js 20 for docs sites (#18026) (Milos Djermanovic)8c1b8dd
test: add more tests for ignoring files and directories (#18018) (Milos Djermanovic)60b966b
chore: update dependency @eslint/js to v9.0.0-alpha.1 (#18014) (renovate[bot])c893bc0
chore: updatemarkdownlint
tov0.33.0
(#17995) (Nitin Kumar)c5e50ee
chore: package.json update for @eslint/js release (Jenkins)1bf2520
chore: Split Docs CI from core CI (#17897) (Nicholas C. Zakas)320787e
chore: delete relative-module-resolver.js (#17981) (Francesco Trotta)4926f33
refactor: useObject.hasOwn()
(#17948) (Milos Djermanovic)df200e1
refactor: useArray.prototype.at()
to get last elements (#17949) (Milos Djermanovic)750b8df
chore: update dependency glob to v10 (#17917) (renovate[bot])74794f5
chore: removed unused eslintrc modules (#17938) (Milos Djermanovic)10ed29c
chore: remove unused dependency rimraf (#17934) (Francesco Trotta)903ee60
ci: use--force
flag when installing eslint (#17921) (Milos Djermanovic)17fedc1
chore: upgrade @eslint/js@9.0.0-alpha.0 (#17928) (Milos Djermanovic)cb89ef3
chore: package.json update for @eslint/js release (Jenkins)f6f4a45
chore: drop structuredClone polyfill for v9 (#17915) (Kevin Gibbons)412dcbb
chore: upgrade eslint-plugin-n@16.6.0 (#17916) (Milos Djermanovic)02a8baf
chore: Rename files with underscores (#17910) (Nicholas C. Zakas)c0f5d91
chore: remove creating an unused instance of Linter in tests (#17902) (Milos Djermanovic)3826cdf
chore: use jsdoc/no-multi-asterisks with allowWhitespace: true (#17900) (Percy Ma)a9a17b3
chore: fix getting scope in tests (#17899) (Milos Djermanovic)595a1f6
test: ensure that CLI tests run with FlatESLint (#17884) (Francesco Trotta)c7eca43
chore: update dependency markdownlint-cli to ^0.38.0 (#17865) (renovate[bot])cc0c9f7
ci: bump github/codeql-action from 2 to 3 (#17873) (dependabot[bot])